Add diagram into your project:
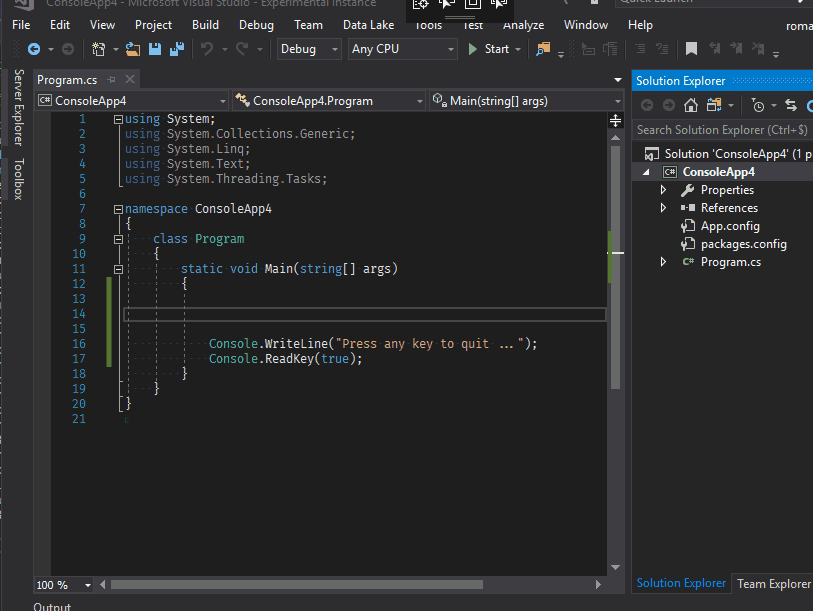
Enter your credentials.
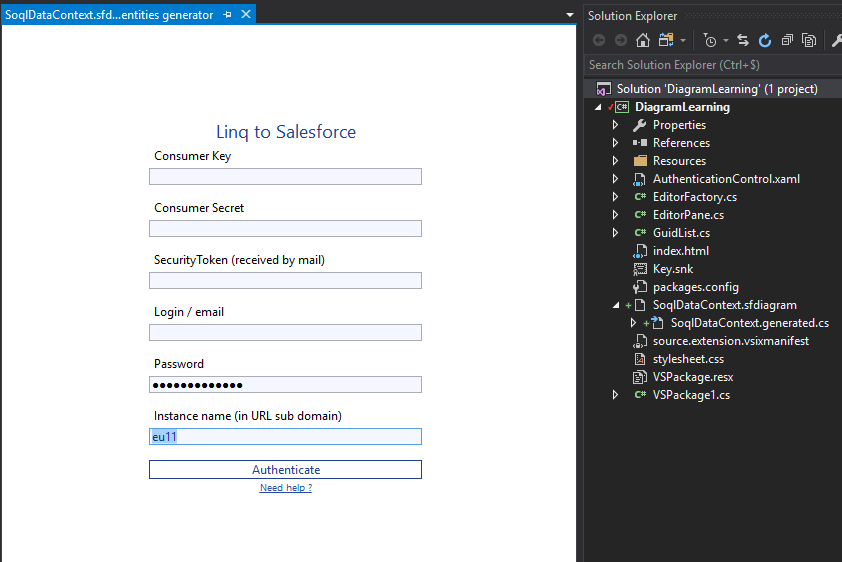
Select entities to generate.
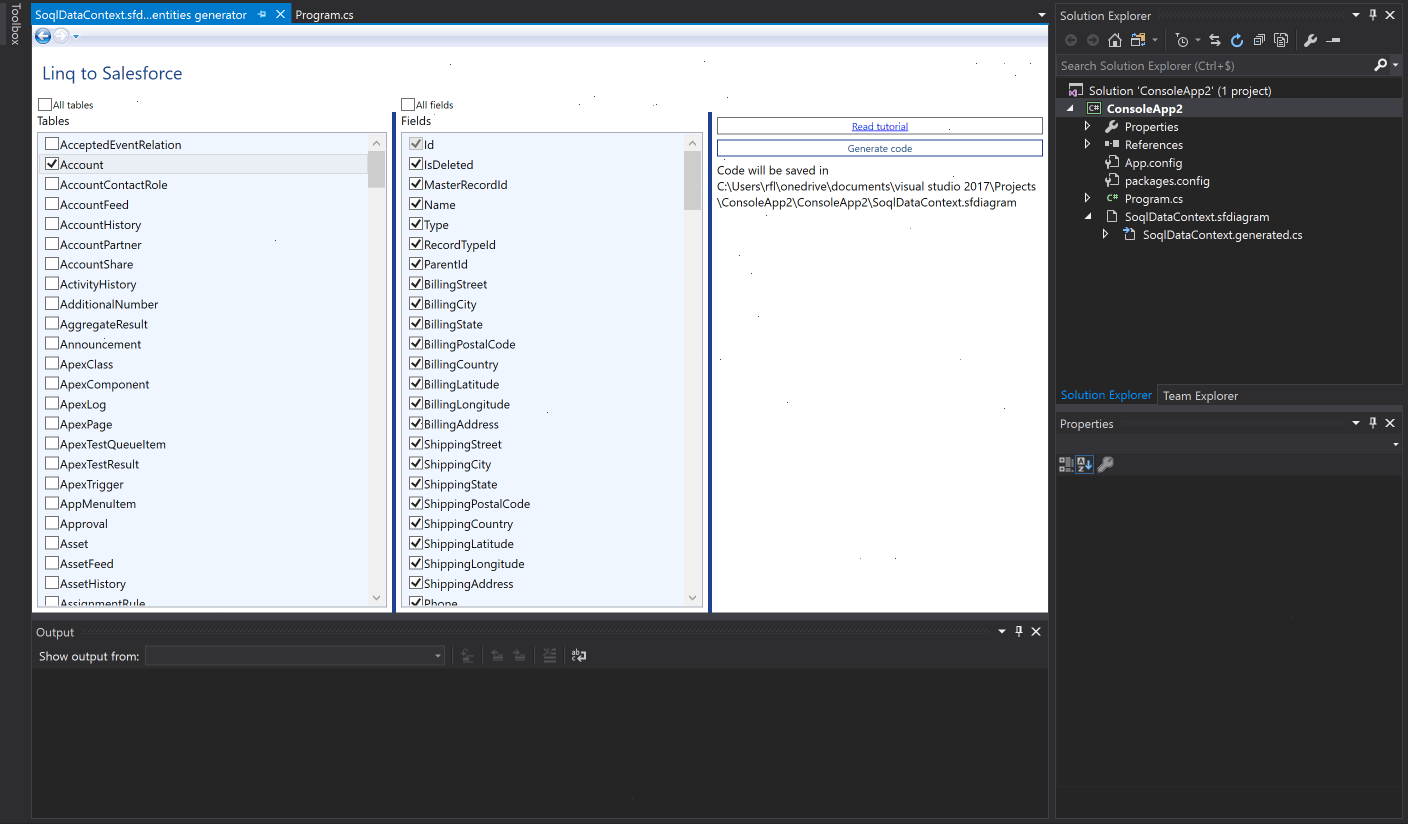
Developing with Salesforce SOQL Linq provider is like a "DB First" entity framework using.
NuGet packages contains 'LinqToSalesforce.ModelGenerator.exe' in tools folder
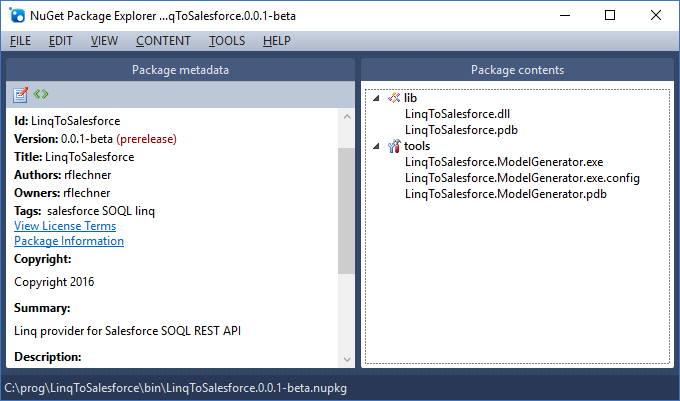
Run it with your credentials in command line:
1:
2:
|
> LinqToSalesforce.ModelGenerator.exe --clientid ... --clientsecret ... --securitytoken ... --login ...
\ --password ... --instancename ... --outputfile "absolute_path_to\Models.cs"
|
Your 'Model.cs' should have things like:
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
11:
12:
13:
14:
15:
16:
17:
18:
19:
20:
21:
22:
23:
24:
25:
26:
27:
28:
29:
30:
31:
32:
33:
|
public class Account : ISalesforceEntity
{
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = null)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
protected bool SetField<T>(ref T field, T value, [CallerMemberName] string propertyName = null)
{
if (EqualityComparer<T>.Default.Equals(field, value))
return false;
field = value;
OnPropertyChanged(propertyName);
return true;
}
private System.String __Id;
[EntityField(false)]
public System.String Id
{
get { return __Id; }
set { SetField(ref __Id, value); }
}
private System.String __Name;
[EntityField(false)]
public System.String Name
{
get { return __Name; }
set { SetField(ref __Name, value); }
}
}
// etc ...
|
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
11:
12:
13:
14:
15:
16:
17:
18:
19:
20:
|
using LinqToSalesforce;
using static System.Console;
var impersonationParam = new Rest.OAuth.ImpersonationParam(clientId, clientsecret, securityToken, username, password);
var context = new SalesforceDataContext("eu11", impersonationParam);
var accounts = (from a in context.Accounts
where !a.Name.StartsWith("Company")
select a).Take(10);
foreach (var account in accounts)
{
WriteLine($"Account {account.Name} Industry: {account.Industry}");
foreach (var contact in account.Contacts) // lazy load contacts
{
WriteLine($"contact: {contact.Name} - {contact.Phone} - {contact.LeadSource}");
foreach (var @case in contact.Cases) // lazy load contact's cases
WriteLine($"case: {@case.Id}");
}
}
|
SoqlContext contains an entity tracker.
So you can update your entities with:
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
11:
12:
|
var accounts = from a in context.Accounts
where a.Name.StartsWith("Company")
select a;
foreach (var account in accounts)
{
var newName = $"{account.Name}_{DateTime.Now.Ticks}";
WriteLine($"Account {account.Name} renamed to {newName}");
account.Name = newName;
}
context.Save(); // entities are detached from the tracker and saved on the server
|
SoqlContext contains an "Insert" method.
When you use it, the entity is directly sent to Salesforce and attached to the tracker.
So you can change properties after inserts.
1:
2:
3:
4:
5:
|
for (var i = 0; i < 100; i++)
{
var account = new Account { Name = $"Company {i}" };
context.Insert(account);
}
|
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
|
var accounts = from a in context.Accounts
where a.Name.StartsWith("Company")
select a;
foreach (var account in accounts)
{
context.Delete(account);
}
context.Save();
|